Hello guys, so in this article, I would be writing about how to perform Benchmarks in your C# console application.
The good thing about this implementation is that it is super easy.
A benchmark could be described as a series of test used to compare the performance of different code implementations based on a particular criterion or different criterias.
For Example I have this class below:
public class Loops
{
private readonly int[] _values;
public Loops()
{
_values = Enumerable.Range(0, 100).ToArray();
}
public int ForLoopWithArray()
{
var total = 0;
for (var i = 0; i < _values.Length; i++) total += _values[i];
return total;
}
public int ForEachLoopWithArray()
{
var total = 0;
foreach (var i in _values) total += i;
return total;
}
}
So in the code above I want to see if the ForEachLoopWithArray method is faster than the ForLoopWithArray method.
You could possibly just add a timer before code execution and calculate the difference between time before code execution and when execution stops. But that won’t be very accurate due to it isn’t tested several time. You could then decide to create a more accurate benchmark by executing the code multiple times, finding the mean and standard deviation of all the runs and comparing it to the code execution of the relative code.
But instead of going through all these hassle. There is a really cool DotNet library called BenchmarkDotNet 🥂🥂
You can add the BenchmarkDotNet NuGet to your project using the dotnet cli command:
dotnet add package BenchmarkDotNet
You can check this link to see other ways of doing that.
BenchmarkDotNet allows you to easily attach an attributes (Benchmark attributes) to the methods you want to test or compare. And that is all you need to implement the magical wonders of BenchmarkDotNet.
Next, all you need to do is to call the Run method in the BenchmarkDotNet class using the name of the class containing your test methods as the its Generic Type.
The code below shows my complete code using the BenchmarkDotNet library to run the Benchmark.
// See https://aka.ms/new-console-template for more information
using BenchmarkDotNet.Attributes;
using BenchmarkDotNet.Running;
BenchmarkRunner.Run<Loops>();
public class Loops
{
private readonly int[] _values;
public Loops()
{
_values = Enumerable.Range(0, 100).ToArray();
}
[Benchmark]
public int ForLoopWithArray()
{
var total = 0;
for (var i = 0; i < _values.Length; i++) total += _values[i];
return total;
}
[Benchmark]
public int ForEachLoopWithArray()
{
var total = 0;
foreach (var i in _values) total += i;
return total;
}
}
Note :- The code above uses c# 10 syntax and also ensure that you execute your code in release mode for proper execution and benchmarking
Execution of the code above would take some minutes or seconds based on the computing power of your processor.
After waiting for a while I got the result below :-
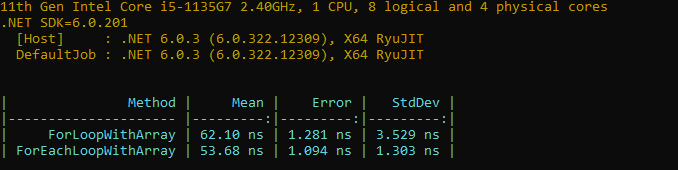
From the analysis, you could see that the ForEachLoopWithArray method performed better in speed during the series of testing
There are also other scenarios that would make a forloop to be faster than a foreach loop in c# and that is where you talk about lowering your C# code and also conversion of your C# code to IL (Intermediate Language) format to understand how the C# compiler sees your code and executes it.
I guess that would be an article for another day 🥂
Thanks for reading through 😜