Hola 🦋, so in this post, I would be talking about using hashes in your URL to identify resources in your database.
Have you ever considered the benefits of using hashed links to numerical autoincremented links?🌚
So some of the benefits include:
- Prevents resource abuse via API calls:- Imagine a user hitting /api/todo/2 because /api/todo/1 returns a resource but when the URL uses hashed identifiers like /api/todo/KEG2lARW the user would have no option but to use the web interface, navigations or controls to obtain the next resource. For instance, by clicking on the next button or a card component of the resource you want to view.
- Still allows you to reserve your auto-incremented integer indexing in your database:- In a relational database, you can easily just convert the unique integer primary key of an entity to unique hashes and transform it back to an integer when a request from a client is made to retrieve the data.
- You don’t have to store hashes in the Database:- Using the library I would be discussing in this article you don’t need to store the hashes in the database as you could execute the hash to integer transformation on the fly for each request.
- Using salts to hash numbers:- To make your hashes unique to your service, you could use a secret key or salt to create your hashes.
One of the popular libraries you can use to make this possible in DotNet 6 is Hashids.
Here is a sample C# script in dotnet interactive exhibiting the use of the Hashids NuGet
#r "nuget: Hashids.net"
using HashidsNet;
Hashids hashids = new Hashids("this is my salt",8);
/* you could encode more than a single integer by comma seperating the integers */
string stringHash = hashids.Encode(4);
Console.WriteLine(id);
int[] numbers = hashids.Decode(stringHash);
/* I could just return the first index since I encoded only a single integer */
foreach(int number in numbers){
Console.WriteLine(number);
}
The salt or secret key should be guarded properly, to avoid easy deciphering of the transformation process behind your hashes.
You could use the library to encode more than one integer by comma-separating integers in the Encode method (eg hashids.Encode(3,4,7).
Next, I would show you how to easily inject this library in your DotNet web api service using Visual Studio🥂
- Open your web project in Visual Studio
- Click on the Tools tab >> select NuGet Package Manager >> select Manage Nuget Packages for Solutions:-
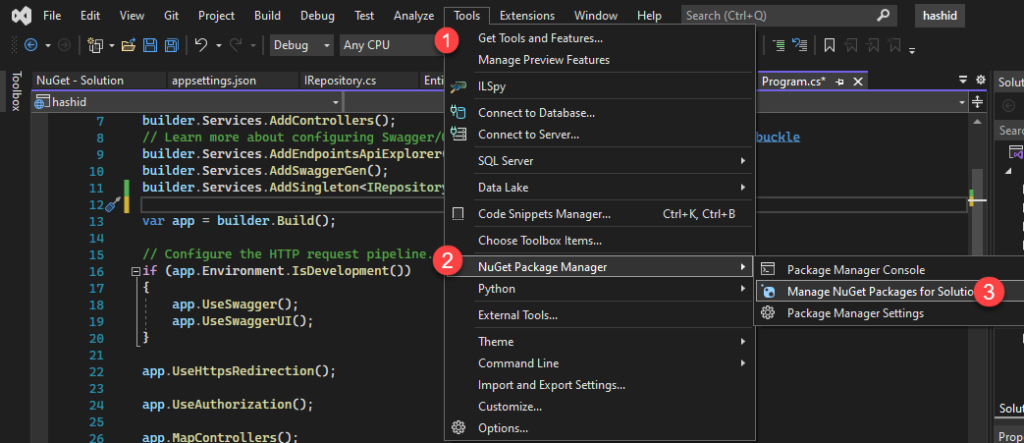
- Type hashids >> Click on the Hashids.net >> Select the desired project to install into >> Install the NuGet:-
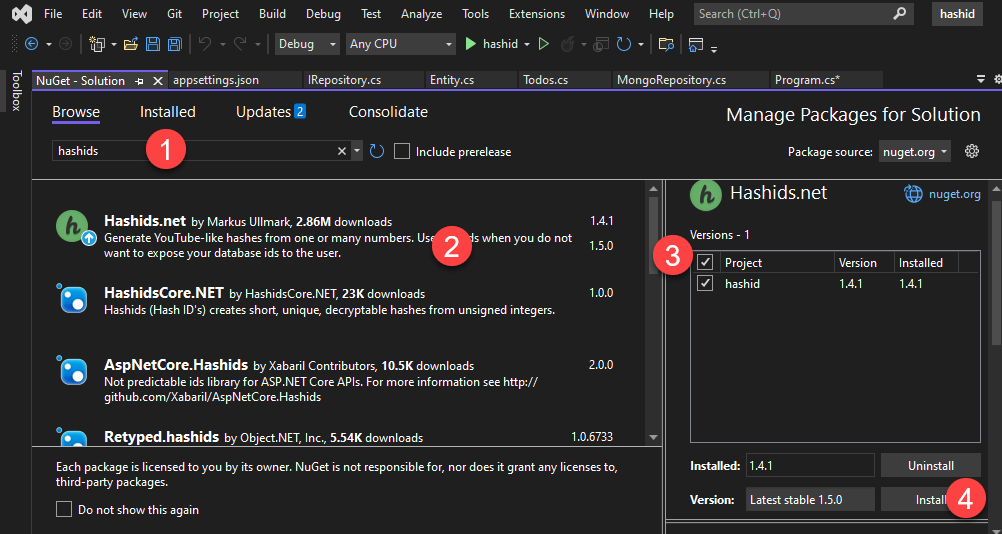
- In Program.cs file add its service via dependency injection ( this article assumes dotnet 6 web API ):-
builder.Services.AddSingleton<IHashids>(sp => new Hashids("Your secret",[minimum hash length]));
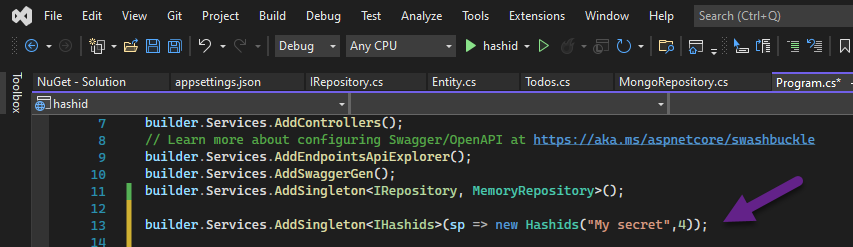
- You can now use the Hashing service in your controller like this: –
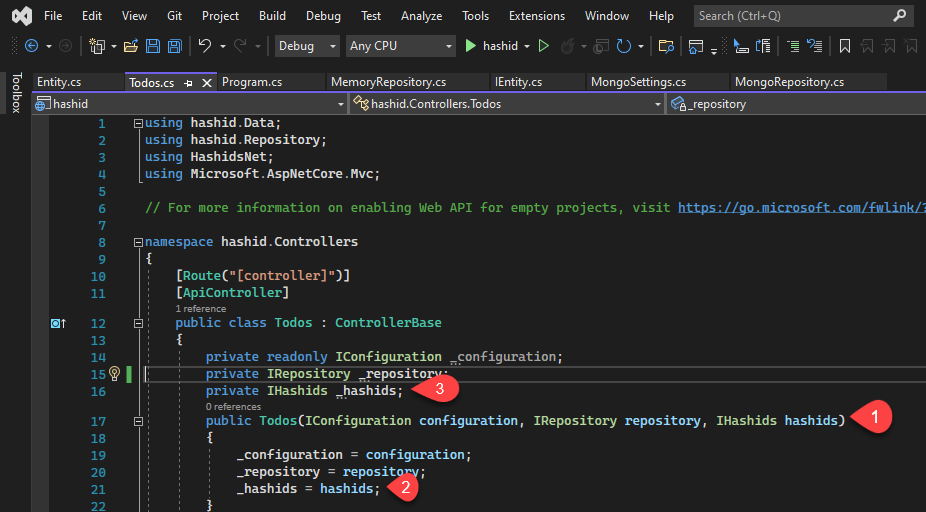
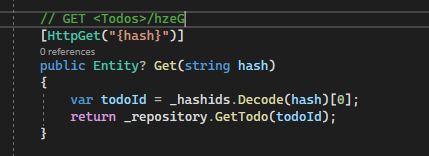
So you’ve come to the end of this article. Hope you found it interesting 🥂.
PS:- I didn’t consider error checking in the code above