Hello peeps, so in this writeup, I would be talking about the serverless integration of the Prisma node library with Azure functions.
Prisma is an awesome node tool that allows users to easily query the database. Currently, it has database support and bindings for MySql, PostgreSQL and SQLite. Prisma makes rest API and GraphQL integrations in your backend seamless.
Prisma is a smart library that is built dynamically by querying your database and building its own client API or by making migrations to the database using its Schema file.
Prisma has lots of features which I won’t be able to talk about in this post but rather focus on how to make use of it in your serverless Azure function.
In this post, we would be building a todo list API using Azure function and a MySQL database. The Azure function would be running on a windows environment.
The Azure portal provides various ways of deploying your functions but in this post, we would be making use of the Vscode method of deployment using Vscode extensions.
Before getting into Vscode, run “npm install -g azure-functions-core-tools”. This is a CLI tool that would help in bootstrapping the templates needed for your function to work.
The following extensions would be needed in Vscode
- Azure Account
- Azure Functions
- Azure Database (not really necessary if you have an existing database server)
To continue, make sure you have signed-in to your Azure account using the Azure Account extension.
Once you are done with that, create a folder, open your terminal in the newly created folder directory and run “func init”. This would display various runtime options.
Since we are using node in this post, continue by selecting node, after that, select javascript in the next option that would be displayed and wait for your the command to set up some necessary files for your function app.
Next, run “func new” in your terminal this would display various options for you to select from as triggers to your serverless function.
Select the HTTP trigger and use “create-list” as the function name.
In no time, the create-list folder would be created. Navigate to the create-list folder and open the index.js file, clean the template code in it and paste this:-
Then open the function.json file and change the auth level property in the binding array to “anonymous”.
Next, create three more functions using the “func new” command. Name each of them “read-list”, “update-list” and “delete-list” respectively using the HTTP trigger.
Below are the following files that would be used for the various index.js files of the created folders( read-list, update-list and delete-list)
For each of the folders created, change the authLevel property in the bindings array to anonymous in the function.json file. This would allow us to make a request to the Azure function without the need for an API key.
Once you’re done with that, run “npm install @prisma/cli @prisma/client” in your terminal to install the Prisma dependencies.
Please do note that you can install the “@prisma/cli” as a development dependency but don’t do that for the “@prisma/client” as it would be removed during the npm pruning process which would lead to bugs after deployment since that’s the main dependency that is required in the production environment
Next, run “npx prisma init” to initialize the Prisma folder which would have the “.env” and the “schema.prisma” file.
Once the files have been created, open the “schema.prisma” file and change it to:-
Ok, let me explain some things here.
By default, Prisma generates its javascript client library, typescript type definitions and query engine in the “.primsa/client” folder under the npm_modules directory.
During deployment, Azure function doesn’t copy the node_modules because it could be quite large, rather it makes use of the package.json file to run an npm install command once it has been deployed.
Due to this, we would need to run another “npx prisma generate” command to populate the Prisma client library. To avoid this we can generate the client library in a folder outside the npm_modules locally in Vscode.
In the code above in the generator client block, I set the output to a “client” folder under the “prisma” directory.
Since we would be making use of a windows environment I also set the ” binaryTargets” variable to “windows”. This would download the required query engine for windows.
Next, create your MySQL server, disable SSL options as that won’t be needed in this tutorial.
Run the query below to generate your table:-
Get the MySQL details below to set up the connection in your “.env” file under the “prisma” folder:-
- The user name on your MySQL server
- The hosting address on your MySQL server
- The password on your MySQL server
Once you’ have gotten the details above, open the “.env” file under the “prisma” folder and attach your MySQL URL to the DATABASE_URL variable like this:-
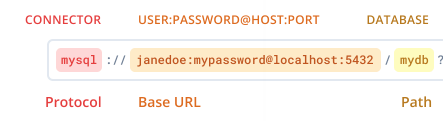
In your terminal run “npx prisma introspect”. This command would create the Prisma schema from your database. open your “schema.prisma” file and you should see this:-
Next, run “npx prisma generate” this would create the prisma client library using your prisma schema.
You can run “func start” to test your functions locally. After running the command wait a little bit for the server to start and you should see the created API endpoints in your terminal.
It would probably look like this:-
You can use PostMan or any client of your choice to test the endpoints
The create-list endpoint requires a POST request with body that has a JSON object with a todo property
The read-list endpoint uses the GET request and doesn’t require a body in its call
The delete-list endpoint requires a POST request with body that has a JSON object with an id property.
The update-list endpoint requires a POST request with a body that has a JSON object with an id property that is assigned to the id of the todo you want to update and also a todo property that is assigned to the updated todo.
Next, click on the Azure icon on Vscode, after that click on the upload option arrow icon in the Azure function section.
You should see the name of the function app that was made locally.
Click on it, select the existing subscription you have on azure.
Next, click on create new function app in azure, type in the name of preference for your azure app, the name would be used to generate the link in this format “<function app name>.azurewebsites.net”.
Select “node” as the runtime, select the location of preference for the resources and wait for your function app to be deployed,
click on initialize app for use with vs code
In case you see an option of deployment using either Linux or Windows, select the windows option. Wait for it to be deployed and your serverless function should be up and running.
Go to your Azure portal and test the API’s
You can clone my GitHub repo for this post here. Remember to insert the MySQL link in the “.env” file under the prisma folder to yours.
To learn more about the Prisma library you can check out the docs here and for the Azure functions you can check it out here.
Thanks if you read up to this point. If you have any question, please feel free to leave it in the comment section, you can also drop your contribution or correction there as well.
No Responses