Hola folks 👋👋👋😎😎😎.
I’m back and banging, smoking hot 🔥….just kidding.
So have you ever wondered how to use GitHub API in your CLI application without copying your access token manually from GitHub Developer Settings🤔🤔….tick tock tick tock. 🕰️🕰️
If you’re familiar with the OAuth web-flow you may decide to create a server that runs in your CLI tool waiting to receive the access token from a web redirect but why would you want to set up a server in your CLI tool just to receive access tokens😢 and that is where you have the luxury of utilizing GitHub Device flow to retrieve access tokens.
So let me get into the working mechanism behind GitHub OAuth Device Flow 🚀🚀🚀
The GitHub Device Flow
The GitHub Device flow is an authentication process that allows you to retrieve access tokens in a browserless environment (ie terminal or daemon) securely.
Working Mechanism of the GitHub OAuth Device Flow:
1. Registration Stage: This is the stage where you register your CLI application that would be consuming the GitHub API. You can register a new application by clicking here.
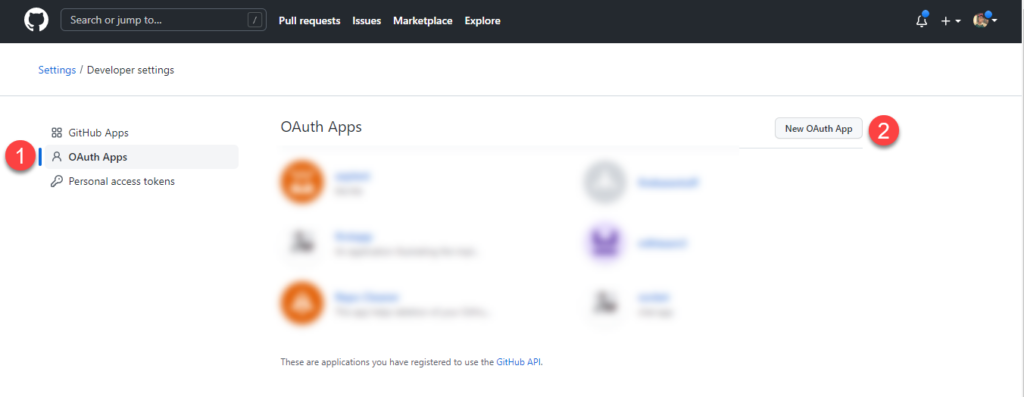
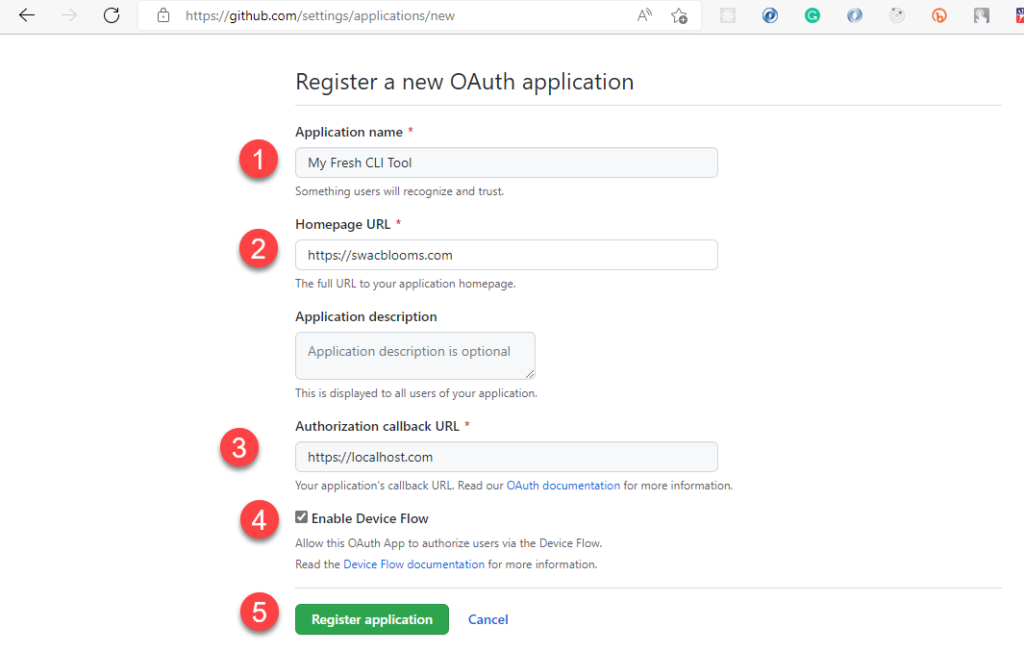
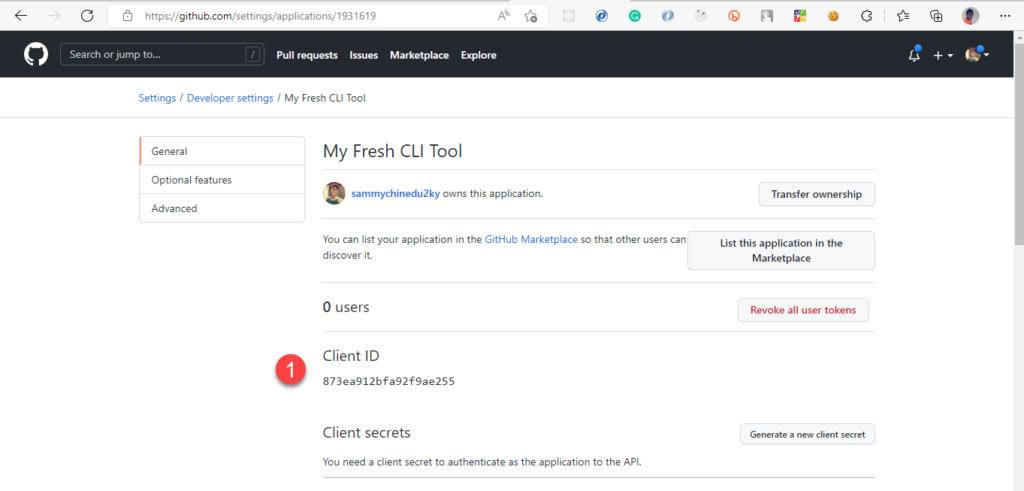
During the registration process, the Authorization Callback isn’t that important, because we are working with the terminal or headless application. So you could use any Authorization Callback URL of your choice. Also, do ensure that you tick the “Enable Device Flow” checkbox.
2. Retrieval of user and device code stage: In this stage, you would have to make a POST request to the endpoint below
POST https://github.com/login/device/code
The POST request above requires the following input parameters:
– client_id: This is gotten after registering your application on GitHub.
– scope: This is used to define the associated permission needed in your tool.
I usually add the Accept header of the POST request set to “application/json” so that the response would be retrieved in a JSON format.
The JSON response contains some important data like: “device_code”, “user_code”,”verification_uri”,”expires_in” and “interval”.
From the response, you would need to open a tab in your browser using the “verification_uri”. The webpage would require a “user_code” which you can retrieve from the response of the POST request above.
3. Retrieval of the access token stage: After opening the “verification_url” in your browser, your CLI tool should be polling the endpoint below until it retrieves a payload containing the access_token.
POST https://github.com/login/oauth/access_token
The request should be made with the following input parameters: “client_id”, “device_code” and “grant_type” which must be set to “urn:ietf:params:oauth:grant-type:device_code”.
The “device_code” should be retrieved from the response of the initial POST request.
Also, ensure you don’t request for the access_token below the minimum polling interval which should be retrieved from the response of the initial POST request.
And hola you would have your access token which you can use to do all sorts of GitHub API manipulation within your set scope 😎🚀. That how easy it is.
So I implemented this flow using C# and also added some extra features like automatically opening your browser (used Microsoft edge in my code) and also automatically adding the user_code to your clipboard 😅.
Below is my code that implements the OAuth Device flow for GitHub. It basically retrieves your access token and uses the access token to create a repository with the aid of the Octokit library:
I used the code below to automatically open a new tab in Microsoft Edge using the verification_uri gotten from the first request.
//open browser
Process.Start(new ProcessStartInfo
{
FileName = "Powershell",
ArgumentList = { "Start-Process", "msedge", Convert.ToString(getCodes["verification_uri"]) },
});
From the code above you can see that my application has a dependency on Power shell and Microsoft Edge being available in your computer. You could decide to customize it to use any other browser on your system.
My code doesn’t persist the access token, but you could persist yours in maybe the Windows Registry or any storage location of your choice. This would prevent you from frequently requesting the access token from GitHub.
You can find out about GitHub OAuth Flows here and my repository here.
Thanks for reading and I hope you enjoyed it 😅.